ocd.storage
– Storage classes¶
This module contains the classes that act as a storage for data needed by OCD to take decisions.
-
class
ocd.storage.
OCDVault
(conf)[source]¶ Bases:
object
The Vault is handed events and knows which one it can store and where. Each variable is stored into a
Container
instance, or child thereof, and all the instances are exposed as attributes.Parameters: - conf :
pyhetdex.tools.configuration.ConfigParser
configuration object
Attributes: - conf
same as input
topics
Returns the list of topics the vault can handle
container_names
Return the list of container names
-
create_containers
(self)[source]¶ Generator that yields the containers, their names, the topics they handlers and the topic handlers.
Yields: - container_name : string
name of the container
- container :
Container
container that stores the information
- topic : string
name of the topic handled by the container
- handler : callable
function that handle the topic. Typically is
ContainerEventMeta.handle_event()
-
store_event
(self, topic, event)[source]¶ If the topic is relevant, call the functions/methods to store the event.
Parameters: - topic : string
topic of the event
- event : dict
event to handle
Returns: - bool
whether the event has been handled or not
-
topics
¶ Returns the list of topics the vault can handle
-
container_names
¶ Return the list of container names
- conf :
-
class
ocd.storage.
MetrologyVault
(conf)[source]¶ Bases:
ocd.storage.OCDVault
Vault containing metrology data.
Examples
>>> from ocd.config import load_config >>> from ocd import tcs_proxy >>> conf = load_config() >>> tcs_proxy.init(conf) >>> ocd_vault = MetrologyVault(conf) >>> ocd_vault.store_event('pas.Guider1.metrology_data', ... {'fit.gauss_par(3)': 42, ... 'fit.gauss_par(4)': 42, ... 'filter.magnitude': 42, ... 'photometry.fixed_skymag': 42, ... 'photometry.kron_skymag': 42, ... 'photometry.fixed_mag': 42, ... 'photometry.kron_mag': 42, ... 'image_quality': 1.2, ... 'transparency': 0.8, ... 'plate_scale.x': 0.196, ... 'plate_scale.y': 0.196, ... '__data_time': 43, }) True >>> # get the Container instance for the fwhm quantity >>> fwhm_container1 = ocd_vault.fwhm_g1 >>> fwhm_container2 = ocd_vault['fwhm_g1'] >>> fwhm_container1 == fwhm_container2 True
All the relevant metrology values from the
pas.Guider{1,2}.metrology_data
are stored, however if the values of any of the following keys istrue
the value is masked:photometry.object_at_image_border
photometry.object_in_bad_image_region
photometry.star_ambiguous
photometry.star_not_found
photometry.unreliable_background
Todo
fix the
transparency_g*
description when the missing documentation arrive. See alsoContainerTransparency
.Attributes: - fwhm_g1, fwhm_g2 :
ContainerFWHM
converts the values of
object.fwhm
to arcsec usingplate_scale.x
andplate_scale.y
from thepas.Guider1.metrology_data
andpas.Guider2.metrology_data
event respectively and store it- skymag_g1, skymag_g2 :
ContainerEventKey
store the values of the sky magnitude from the
pas.Guider1.metrology_data
andpas.Guider2.metrology_data
event respectively. The name of the key is defined by thephotometry_skymag
option of the[containers]
section of the configuration- transparency_g1, transparency_g2 :
ContainerTransparency
compute and store the transparency from the
pas.Guider1.metrology_data
andpas.Guider2.metrology_data
event respectively. It uses the star magnitude,filter.magnitude
and illumination correction keywords. The name of the star magnitude is defined by thephotometry_trans
option of the[containers]
section of the configuration; the illumination correction is set to0.86
and can be modified using theillumination_correction
option.
-
create_containers
(self)[source]¶ Generator that yields the containers, their names, the topics they handlers and the topic handlers.
Yields: - container_name : string
name of the container
- container :
Container
container that stores the information
- topic : string
name of the topic handled by the container
- handler : callable
function that handle the topic. Typically is
ContainerEventMeta.handle_event()
-
_container_event_key
(self, event_key, mask_func=None)[source]¶ Instantiate a
ContainerEventKey
and returns it.Parameters: - event_key : string
name of the key in the event whose value must be stored
- mask_func : callable, optional
mask function to pass to the ContainerEventKey; defaults to
None
Returns: - container :
ContainerEventKey
-
class
ocd.storage.
AzimuthVault
(conf)[source]¶ Bases:
ocd.storage.OCDVault
Vault for storing azimuth.
Attributes: - azimuth :
ContainerSkipMasked
store the values of
az
, if thesetup
is done ("true"
), from thetcs.root.ra_dec
event. Only a few azimuth values are retained by OCD, as only the latest one is used.- azimuth_tmp :
ContainerSkipMasked
store the values of
az
, if thesetup
is not done ("false"
), from thetcs.root.ra_dec
event. Only a few azimuth values are retained by OCD, as only the latest one is used. This can be used as a fallback in case theazimuth
is empty.
-
create_containers
(self)[source]¶ Generator that yields the containers, their names, the topics they handlers and the topic handlers.
Yields: - container_name : string
name of the container
- container :
Container
container that stores the information
- topic : string
name of the topic handled by the container
- handler : callable
function that handle the topic. Typically is
ContainerEventMeta.handle_event()
- azimuth :
-
class
ocd.storage.
Container
(maxlen=50, delta_timestamp=None)[source]¶ Bases:
object
Container to store a list of n values, their time stamps and their mask. Quantities like mean, median and standard deviation are exposed as properties.
Warning
time stamps are assumed to be seconds “since the epoch” as returned e.g. by
time.time()
. All the tests are run assuming this.Parameters: - maxlen : int, optional
maximum length of the underlying storage
- delta_timestamp : number, optional
maximum time difference for the values stored in the class in seconds. It must be a positive number or
None
When adding a new value, discard all the values older thandelta_timestamp
with respect to the value timestamp; ifNone
the timestamp is ignored
Raises: - ocd.errors.OCDTypeError
if
delta_timestamp
is not a number- ocd.errors.OCDValueError
if
delta_timestamp
is negative
Attributes: latest
Returns the last value added.
latest_masked
Returns the last non masked value.
mean
Return the mean of the stored values or
NaN
median
Return the mean of the stored values or
NaN
stddev
Return the standard deviation of the stored values or
NaN
if nomean_masked
Return the mean of the non masked stored values or
NaN
median_masked
Return the median of the non masked stored values or
NaN
if nostddev_masked
Return the standard deviation of the non masked stored values or
-
add_value
(self, value, timestamp, mask=False)[source]¶ Store the new value and time stamp. If a
delta_timestamp
is given any entry older thantimestamp - delta_timestamp
are removedThe code assumes that the values are added with increasing time stamp.
Parameters: - value : number
new value to store
- timestamp : float
timestamp in seconds associated to the value
- mask : bool, optional
whether the value is to be masked or not. It follows the convention of numpy masked arrays. By default no value is masked
-
mean
¶ Return the mean of the stored values or
NaN
if no value is present
-
median
¶ Return the mean of the stored values or
NaN
if no value is present
-
stddev
¶ Return the standard deviation of the stored values or
NaN
if no value is present
-
mean_masked
¶ Return the mean of the non masked stored values or
NaN
if no value is present or the mask is allTrue
-
median_masked
¶ Return the median of the non masked stored values or
NaN
if no value is present or the mask is allTrue
-
stddev_masked
¶ Return the standard deviation of the non masked stored values or
NaN
if no value is present or the mask is allTrue
-
latest
¶ Returns the last value added. Raises an
AttributeError
if no value is found.
-
latest_masked
¶ Returns the last non masked value. Raises an
AttributeError
if no value is found.
-
class
ocd.storage.
ContainerEventMeta
(*args, **kwargs)[source]¶ Bases:
ocd.storage.Container
ABC class that extend the
Container
class adding a method to handle a TCS event and store a value derived from it.Derived classes must reimplement the
value_from_event()
.Parameters: - time_key : string, optional
name of the keyword containing the time stamp. Default:
__data_time
- mask_func : callable, optional
function that receives the event passed to
handle_event()
as input and should returnTrue
if the value is to be masked,False
otherwise. If not given orNone
, no value will be masked- args, kwargs :
positional and keyword parameters passed to the parent class
-
handle_event
(self, event)[source]¶ Call
value_from_event()
to extract a value from the event, get the time stamp and, if required, the mask and save it.Parameters: - event : dict
event to handle
-
class
ocd.storage.
ContainerEventKey
(key, *args, **kwargs)[source]¶ Bases:
ocd.storage.ContainerEventMeta
Implementation of
ContainerEventMeta
class that extract and save a single value for a TCS event.Parameters: - key : string
when handling the event, get the value for the given key and store it
- args, kwargs :
positional and keyword parameters passed to the parent class
-
class
ocd.storage.
ContainerTransparency
(conf, *args, **kwargs)[source]¶ Bases:
ocd.storage.ContainerEventMeta
Implementation of
ContainerEventMeta
class that computes the transparency from a TCS event.On top of the masking described in
MetrologyVault
, this container also masks negative values of thefilter.magnitude
value, on the assumption that if no guiding nor photometry is performed on stars with negative magnitude and that the “unset” value is-9.999
.The illumination correction has been included in the guider fotometry.
Warning
We set the default illumination correction to 1. If necessary this value can be overridden with the
illumination_correction
option of the[containers]
configuration option. This might be eventually removed.Parameters: - conf :
pyhetdex.tools.configuration.ConfigParser
configuration object
- args, kwargs :
same as in
ContainerEventMeta
-
value_from_event
(self, event)[source]¶ Calculate the transparency as:
\[\frac{10 ^ {-0.4 * (mag - filter\_mag)}}{illum\_corr}\]where
mag
is the measured magnitude of the star and is stored in the key defined by thephotometry_trans
option of the[containers]
section;filter_mag
is the reference magnitude of the star, as communicated to TCS, and is stored in thefilter.magnitude
key;illum_corr
is the illumination correction for the current position of the tracker; it is set to 1 or the value of theillumination_correction
option of the[containers]
section;
Parameters: - event : dict
event to handle
Returns: - transparency : float
- conf :
-
class
ocd.storage.
ContainerFWHM
(conf, *args, **kwargs)[source]¶ Bases:
ocd.storage.ContainerEventMeta
Implementation of
ContainerEventMeta
class that computes the FWHM in arcseconds as:\[\sqrt{(\sigma_x * pscale_x) * (\sigma_y * pscale_y)}\]where the
sigma_x
andsigma_y
are the x and y standard deviation, in pixels, from the Gaussian fit of the guide star profile (fit.gauss_par(3)
andfit.gauss_par(3)
) andpscale_x
andpscale_y
are the pixels scale (plate_scale.x
andplate_scale.y
).If either
fit.gauss_par(3)
orfit.gauss_par(4)
is zero or negative, the value of fwhm is masked.Parameters: - conf :
pyhetdex.tools.configuration.ConfigParser
configuration object
- args, kwargs :
same as in
ContainerEventMeta
- conf :
-
class
ocd.storage.
ContainerSkipMasked
(key, *args, **kwargs)[source]¶ Bases:
ocd.storage.ContainerEventKey
Container to store only values that are not masked.
-
add_value
(self, *args, **kwargs)[source]¶ This method calls
Container.add_value()
ifmask
isFalse
. Otherwise does nothingParameters: - all :
-
Inheritance scheme¶
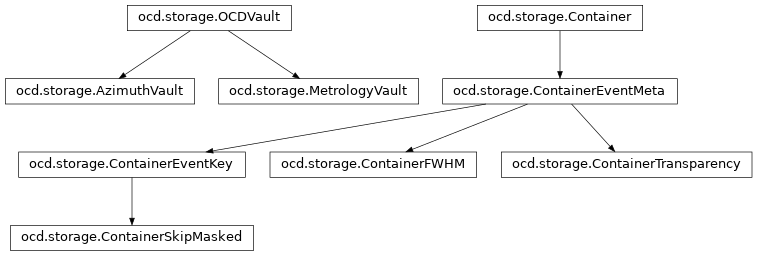